Blog > The Ideal Approach to Teach Mobile Development in MDB
The Ideal Approach to Teach Mobile Development in MDB
Crafting curriculum to teach software development
2,731 words, ~ 14 min read
mdb
teaching
For the past year, I have been the React Native Instructor in Mobile Developers of Berkeley, a club at UC Berkeley.
In this time, I've had to think a lot about what it means to learn software development and how to create curricula that is approachable yet complete across a variety of rapidly-changing paradigms.
What follows is a collection of thoughts loosely assembled while I was on a flight without internet access, before I realized I could watch movies on the airplane WiFi.
important note: as always, this is my personal view, unendorsed by anyone or anything.
TL;DR
My view is that in this particular context, the ideal pedagogy is to provide a smaller degree of hands-on experience in favor of project-driven development, avoiding "tutorial hell" and building skills extending to any form of software.
Table of Contents
- Background
- What is the goal of the training program?
- What is the structure of the training program?
- Fin
Background
I'm including a section on my personal background with learning software development to account for potential biases in my perspective.
I came into college without much experience. I had taken AP CS A in high school, but I understood virtually nothing (on the AP exam, I had written pseudocode for nearly all of the questions).
I struggled quite a bit in my first few CS classes, mainly due to a lack of exposure. Concepts like tree recursion were baffling, and the technical explanations were often more confusing.
But once I got used to the patterns within these classes, I was able to adjust quite well. However, learning other parts of software - such as React - was still very, very challenging for me.
I learned React Native from when I was a newbie in MDB's training program back in Spring 2021, the first semester it was offered. As the instructor, my task is to teach, of course, but also to expand on the program. To do so, I needed to reflect on what I wanted to accomplish (the goal) and how to accomplish it (the structure of the program).
What is the goal of the training program?
Classes
Consider CS 61B, a data structures class. The goal is to teach students to think about tradeoffs. For example, using an array allows constant access time, but using a linked list allows dynamic sizing.
It also extends to the algorithms section of the course, where there can be one task (e.g. how to get from location A to location B) with numerous methods to solve (BFS, DFS, Dijkstra's, Bellman-Ford, A*) based on the information available, the goal, and the desired time complexity.
Note that this isn't necessarily unique to any particular class. Other classes, like CS 170, a class on efficient algorithms and intractable problems, also are about tradeoffs.
The World of Software Development
In contrast, let's say the goal is to learn software development. For the sake of clarity, we can think of this as the process of what it means to make software consumed by people - coding, architecture, workflows, git, etc.
Few classes at Berkeley directly teach software development (the only one that comes to my mind is CS 169A). Most students garner real-world experience through software engineering internships or work experience.
Here is the classic catch-22 (which applies to a variety of industries). To get such software engineering opportunities, employers like to see past work experience - which in turn requires having the very work experience that is trying to be obtained.
In comparison, software engineering has one key thing that makes it relatively more doable (in my view): projects. If I can make a RESTful API and a frontend for an interesting project, perhaps track some analytics, that's an entry for my resume. Many people I know have simply fantastic projects they're able to discuss for hours, directly leveraging into more experience. Specific discussion about the nature of CS projects and recruiting could be its own post, so I'll leave it at this.
The issue remains: making projects is hard, since it still requires some notion of understanding software development. Being able to deploy an API and a frontend to consume it is tricky, and it's not as straightforward as it sounds.
MDB
So where is MDB in all this?
In my view, MDB is a student organization whose goal is to empower students to learn about software development.
One element of this is doing things to help with recruiting to get students experience in the real world. We do resume reviews, mock interviews, and answer all sorts of questions to help clarify a challenging process.
But let's consider the training program for a moment. The goal could be as simple as: "teach React Native", since after all, it is the React Native Training Program and we are a mobile development club.
For one, this isn't very useful. Teaching just React Native means that people can build cross-platform mobile applications (and web, since React Native uses the same concepts found in React). Perhaps this includes understanding how to make API calls on the frontend as well, so it effectively teaches frontend development.
To take a step away - consider writing code in C. Being able to understand the patterns of the C compiler into the underlying Assembly files makes you a better C developer, since that one extra level gives you insight into how you should structure your code. As an example - consider loop unrolling, which reduces potential branch checks and jumps.
The same applies to frontend development. To be a better frontend developer, it makes sense to learn backend development and some information about database management.
Most importantly, MDB is chiefly a club trying to build solutions to problems. It just so happens that mobile is the means through which that happens (something I've repeated at many, many info sessions). It means that we are a software development club, building mobile solutions.
One last note - the emphasis on conceptual learning.
There are a few considerations here. For one, consider how fast things change. It was only in the 90s we saw the web, the 2000s we saw jQuery, and the 2010s we saw React and other frameworks. Even now, there are so many things being released - Next.js, for one, brings server side rendering for increased performance, other JavaScript runtimes like Bun or Deno, etc. - the list goes on and on. I'd wager that in just a few years, the world of software development looks vastly different.
Underlying concepts, though, remain the same. Some new concepts are introduced, which can be learned.
What do I mean by concepts? I mean language-agnostic paradigms. Consider learning the nuances of TypeScript - the specific syntactic sugar unique to the language. Compare this with learning the concept of state (state as a minimal representation of a system, not state like Florida). State is independent of any language, in fact, it's independent of software - I first learned about state in control theory. But the specifics of TypeScript, such as Pick and Omit, are not applicable to Python, Java, C, etc.
That doesn't mean that the nuances of TypeScript are irrelevant. Since so much of frontend and backend development is in TypeScript, I think it's quite valuable to know Pick and Omit to make better types and thus catch more bugs with the compiler instead of after deployments. It just means that learning state as a concept that will persist.
The takeaway - our goal is to teach concepts. The concept of state might not make much sense without understanding the syntax of the useState hook, so there will be some element of language-specific paradigms, but that's fine - it is merely a means to an end.
This distinction between concepts and syntax isn't going to be very relevant; in fact, the first lesson in the training program is purely JavaScript syntax. But it means that things are always structured as motivation, concept, implementation, example, project (to be described in more detail below).
What is the structure of the training program?
Topics Covered
At a surface level, the training program covers the following (in roughly this order):
- Dev Environment Setup
- JavaScript Basics
- React and React Native Basics
- React Navigation
- Promises, Async/Await
- Firebase Firestore
- Firebase Cloud Storage
- TypeScript
- Systems Design
- Firebase Authentication
- Design
- Redux
- Backend
- Web
It's a lot.
I'll be the first to say that it's not possible to learn all of these topics in significant depth in just the span of a few weeks. In fact, this is all part of a 7 week training program.
But that's not the goal. The goal is primarily for newbies to be able to build apps, which they can do with TypeScript, React Native, React Navigation, and Firebase.
However, my hope is that if someone down the line sees the term "load balancer" somewhere down the line, they can remember hearing about it and then a quick refresher should get them up to speed. The same applies for Redux, more complicated backend functions, and even web design (web is a special case, that I'll expand on below).
Introducing Concepts, Not Syntax
There's a rough pattern that I like to follow in all of the training program lectures:
- Motivation
- Concept
- Implementation
- Example
- Project
This is best illustrated by a sample concept: state. Note that the motivation, concept, and implementation are verbal with the use of slides, the example is a demo done live, and the project is done by newbies separately.
Motivation
Let's say we book a room, for example, Chou N155. We hold an event, and at the end, we want to return it as we found it in the hopes of being able to book the room again in the future. How can we record the information of how the room was at the start?
We could take an endless amount of pictures, but we want to avoid having too much unnecessary information.
Concept
Introducing state, a minimal representation of a system at a given moment of time. Note the use of minimal - state usually will track things that we expect to change that we would like to keep track of.
Implementation
React allows us to "hook" into the state functionality through useState
, which gives us a state object and a function to update the state.
Example
In hackshop (our weekly meetings), we built MDSlack - a simple version of Slack, where we can modify and update state in viewing channels.
Project
The first mini-project in the training program requires implementing the state of a game to monitor the score, selected options, random generation, etc.
Changes to Topics Covered
With the rapid pace of software changing, it's inevitable for code syntax to change and lessons to be updated. One major one was the switch from Firebase v8 to v9. In v8, imports looked like the following:
import firebase from 'firebase'
import 'firebase/app'
import 'firebase/firestore'
Whereas in v9, the structure is the following:
import { getDoc, setDoc } from 'firebase/firestore'
In v8, access is done through the firebase object imported in, whereas in v9, access is done through the use of specific functions being imported. For any training program to stay relevant, these have to be updated.
What's more complex is changing the underlying concepts being covered. There are two notable changes that I've brought about: adding systems design, and adding web.
Systems Design
This is definitely one of the areas that is above and beyond for the training program. Many new grads are not even asked systems design questions due to the lack of real-world experience. That being said, I have found it useful to know some of the basics of systems design just for context when learning or discussing other topics. Most of all, there's a good chance real-world software development involves some component of systems design, and I wanted newer members to be exposed to these kinds of concepts early on.
Note that this is not formal systems design training that you might find online or in books, merely a primer to the primers in the sense that we talk about some concepts like the CAP theorem or different ways of partitioning data.
In demos, this is lightly covered; after doing initial demos in React and React Native mimicking Slack in a project called MDSlack, I launched a larger project based on Spotify. This featured a database, a backend, and a lot more complexity being supported, so we started with a backend architecture diagram and used different files to mimic the microservice architecture, with all files at MDSpotify.
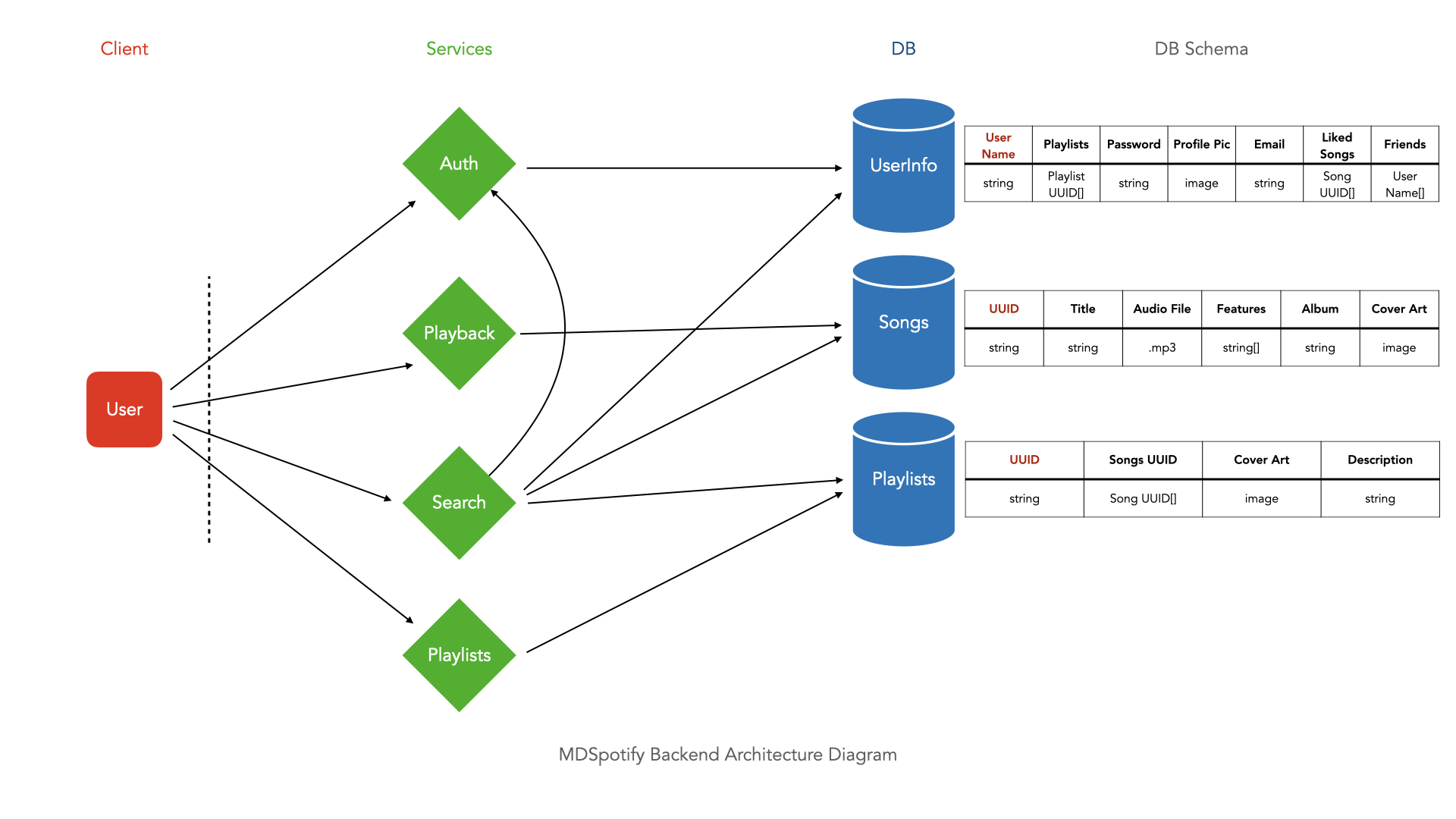
Web
This one might be a bit surprising, since we are a mobile club; why teach web? Well, to be fair, this is more of explaining the analogies between React and React Native. Many jobs in industry are in React, so knowing some React specific things about the web like React Router could prove useful. Further, many of the concepts that were covered - such as navigation, backend, etc. - all still apply.
This one is also personally motivated. The summer after I did the training program in React Native, I taught myself React and built a web app. I found that the concepts were very similar, and I wanted to share a few of the pitfalls that I had run into with others.
Demos
There exists a gap between knowing and understanding. It's one thing to hear about state as a concept, and another to be able to take an idea, think about what elements need to be encoded in state (as well as how and why), then actually build out the application.
That's where the demos come in. Going directly into projects is challenging, so this serves as the gateway where I as an instructor can guide the newbies towards what we are implementing.
It's also a chance for me to learn and sharpen my skills. I'll usually come into hackshop with slides ready, go through them, and then ask a general question about what people would like to see included in the demo. But since I don't know what the specifics of what the demo will entail, it requires me to think on my feet for solutions and often debug live.
At the second hackshop, for example, the app suggested was Slack - so we built MDSlack, with a focus on using state. The following week, we built Slack using React Navigation, going over to the mobile world.
Then we switched entirely again to a new suggestion, this time Spotify, doing some systems design, making MDSpotify. Then in future weeks, we built out different features - starting with designing and creating some backend endpoints, then incorporating Redux, and then some authentication (and potentially more!).
In most demos, the demonstrator will have done the demo themselves, know the common pitfalls, and guide the audience towards where they are looking to go.
In this case, I ask questions more broadly, willing to hear any answer. My role is to not to provide the content of those answers, but rather to point out any issues that may arise as a result and to help with the implementation in the application. In fact, I had never implemented Redux myself before - I have experience with projects involving Redux, and I understand it conceptually, but it wasn't something I had done myself. So I had to learn it on the fly - a fun challenge.
Additionally, the choice to use one application and then slowly build on it in the demo provides a cohesive understanding of how different elements all play a role together in building more complex pieces of software.
Fin
There's a lot of things I haven't discussed here that have shaped the training program, and there's even more thoughts I have about teaching pedagogy in general. Perhaps they will merit their own posts in the future. For now, I believe this has sufficient coverage for my taste regarding some of the things I've considered as I've updated and modified the training program.
Made it this far? Like what you hear about MDB? Consider applying! We recruit undergraduate students at UC Berkeley in the fall and spring semesters, and our application will be found towards the beginning of the semester at mdb.dev.
Found this interesting? Subscribe to get email updates for new posts.
First Name
Last Name
Previous Post
Building Apps, a Workshop at CalHacks 9.0